Time Keeping
The ESP32 docs say you can set the RTC to keep time in deep sleep (“RTC clock is used to maintain accurate time when chip is in deep sleep mode”). This post details how to restore normal system time from the RTC in Arduino.
Time keeping is a bit confusing in C. There is my summary:
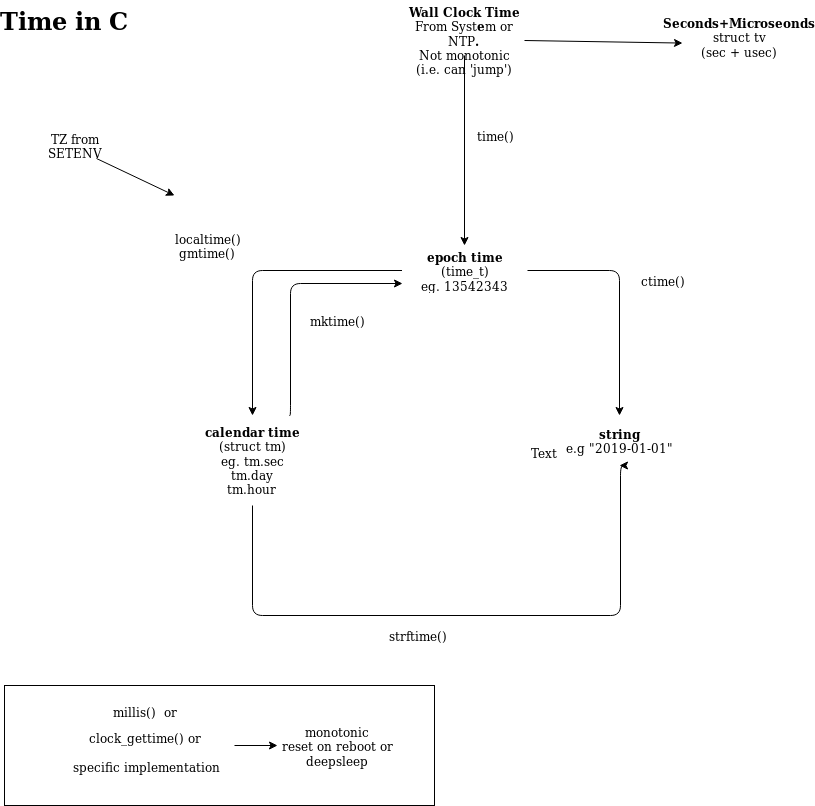
Deep sleep is documented for Arduino. Wall-clock versus system-clock is explained here. Basically: use millis() for loop timing.
Our strategy, to limit WiFi/NTP use, is:
- On reboot, or once daily: turn on WiFi. Use NTP to get the current wall-clock time. This is used for timestamps of measurements.
- On reanimate (i.e. after deep sleep), set the system time by asking the RTC.
- Don’t rely on gettimeofday() or time() to provide monotonic time. Use use FreeRTOS vTaskDelay().
You can use system calls with the ESP32, and the RTC will store and increment time on deepsleep (but not reboot):
settimeofday(&ntpTime,NULL); // set system time
gettimeofday(); // get system time to sec+usec
time(NULL); // get system time to sec
(… I started using “eztime” but it’s heavyweight. The sntp functions of esp-idf are much nicer to work with, and more logical (to my eyes!).
Types
Some hard to find typedefs:
- typedef uint32_t TickType_t;
- int BaseType_t
- time_t uint32_t
- uint32_t millis()